Coding in Crypto: Smart Contracts and Blockchain Development
The rise of blockchain technology has revolutionized financial systems, decentralized applications, and digital ownership. At the core of this transformation is coding, which powers smart contracts, decentralized exchanges, and yield farming protocols like FARM. Let’s explore how coding plays a pivotal role in cryptocurrency and examine key examples of blockchain development.
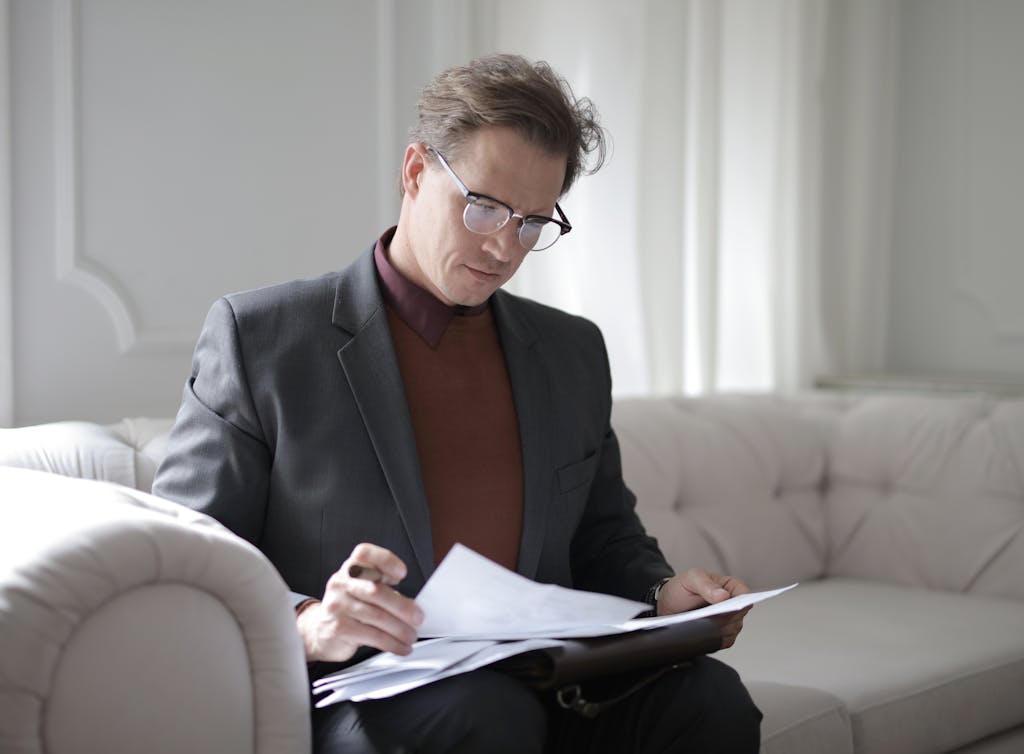
The Role of Coding in Blockchain Development
Coding is the foundation of cryptocurrency networks, enabling automation, security, and transparency. Developers use programming languages like Solidity, Rust, JavaScript, and Python to build decentralized applications (dApps) and smart contracts.
Key areas where coding is essential in crypto include:
- Smart Contracts: Self-executing contracts with predefined rules.
- Consensus Algorithms: Mechanisms like Proof of Work (PoW) and Proof of Stake (PoS).
- Decentralized Applications (dApps): Applications running on blockchain networks.
- Security Audits: Identifying vulnerabilities in smart contract code.
Writing a Simple Smart Contract in Solidity
Ethereum’s smart contracts are written in Solidity, a language designed for the Ethereum Virtual Machine (EVM). Below is a simple Solidity smart contract to store and retrieve a value:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedValue;
function setValue(uint256 _value) public {
storedValue = _value;
}
function getValue() public view returns (uint256) {
return storedValue;
}
}
How It Works:
setValue
stores a number on the blockchain.getValue
retrieves the stored number.- Uses
pragma solidity ^0.8.0;
to ensure compatibility with Solidity versions 0.8 and above.
Building a DeFi Yield Farming Contract
DeFi protocols rely on smart contracts to manage liquidity pools and rewards. Below is an example of a basic liquidity staking contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract YieldFarm {
mapping(address => uint256) public stakes;
mapping(address => uint256) public rewards;
uint256 public rewardRate = 5; // Example reward rate
function stakeTokens(uint256 amount) public {
stakes[msg.sender] += amount;
}
function calculateReward(address user) public view returns (uint256) {
return (stakes[user] * rewardRate) / 100;
}
function claimReward() public {
uint256 reward = calculateReward(msg.sender);
rewards[msg.sender] = reward;
}
}
Key Features:
- Users can stake tokens and accumulate rewards.
calculateReward
computes yield farming rewards.claimReward
allows users to withdraw earned rewards.
The Future of Crypto Coding in 2025 and Beyond
With the growing adoption of blockchain, coding in crypto is set to evolve significantly. Anticipated advancements include:
- More efficient Layer 2 solutions for scaling Ethereum and other blockchains.
- Interoperability protocols enabling seamless cross-chain transactions.
- AI-driven smart contracts for adaptive security and efficiency.
Blockchain development continues to be one of the most sought-after skills in tech. Whether you’re a beginner or an experienced coder, mastering Solidity, Rust, or other blockchain programming languages will be key to building the decentralized future.